Using numpy linspace for effortless array creation
np.linspace(): Create Evenly or Non-Evenly Spaced Arrays
When working with numerical applications in Python, particularly with NumPy, it is common to create arrays of numbers that are evenly or non-evenly spaced. One of the key tools in achieving this is through the use of np.linspace()
.
In this tutorial, we will explore how to effectively utilize np.linspace()
to create arrays with evenly or non-evenly spaced numbers. By the end of this tutorial, you will have a clear understanding of how to use np.linspace()
and when it is more suitable compared to alternative methods.
Creating Ranges of Numbers With Even Spacing
There are multiple ways to create a range of evenly spaced numbers in Python. np.linspace()
is a powerful function that allows you to customize the range based on your specific requirements. Let’s take a closer look at how to use np.linspace()
and compare it with other methods of creating ranges of evenly spaced numbers.
Using np.linspace()
np.linspace()
requires two mandatory parameters, namely start
and stop
, which define the beginning and end of the desired range. Here’s an example:
import numpy as npnp.linspace(1, 10)
The output will be an array of numbers with equal spacing from 1 to 10:
array([ 1., 1.18367347, 1.36734694, 1.55102041, 1.73469388, 1.91836735, 2.10204082, 2.28571429, 2.46938776, 2.65306122, 2.83673469, 3.02040816, 3.20408163, 3.3877551 , 3.57142857, 3.75510204, 3.93877551, 4.12244898, 4.30612245, 4.48979592, 4.67346939, 4.85714286, 5.04081633, 5.2244898 , 5.40816327, 5.59183673, 5.7755102 , 5.95918367, 6.14285714, 6.32653061, 6.51020408, 6.69387755, 6.87755102, 7.06122449, 7.24489796, 7.42857143, 7.6122449 , 7.79591837, 7.97959184, 8.16326531, 8.34693878, 8.53061224, 8.71428571, 8.89795918, 9.08163265, 9.26530612, 9.44897959, 9.63265306, 9.81632653, 10. ])
As you can see, the numbers in the array are evenly spaced between 1 and 10. This is the default behavior of np.linspace()
.
Using range() and List Comprehensions
While np.linspace()
is a powerful tool, there are alternative methods for creating ranges of evenly spaced numbers.
One such method is by using the built-in range()
function and list comprehensions. Here’s an example:
numbers = [x / 10 for x in range(10, 101, 10)]
In this example, the range()
function generates numbers from 10 to 100 with a step of 10. The list comprehension divides each number by 10 to achieve equal spacing.
Using np.arange()
Another alternative is to use the np.arange()
function, which generates a range of numbers using a specified step size. Here’s an example:
import numpy as npnp.arange(1, 11, 0.5)
This will create an array with numbers ranging from 1 to 10 with a step size of 0.5. The output will be:
array([ 1. , 1.5, 2. , 2.5, 3. , 3.5, 4. , 4.5, 5. , 5.5, 6. , 6.5, 7. , 7.5, 8. , 8.5, 9. , 9.5, 10. ])
As you can see, the numbers in the array are evenly spaced with a step size of 0.5.
Customizing the Output From np.linspace()
np.linspace()
offers additional parameters that allow you to customize the output to better suit your needs. Let’s explore some of these parameters.
The start, stop, and num Parameters
In the basic usage of np.linspace()
, the parameters start
and stop
are required to define the range of numbers. Additionally, you can specify the number of equally spaced numbers required within that range by using the num
parameter. Here’s an example:
import numpy as npnp.linspace(1, 10, num=5)
This will generate an array of 5 numbers evenly spaced between 1 and 10:
array([ 1. , 3.25, 5.5 , 7.75, 10. ])
The dtype Parameter for Changing Output Type
By default, np.linspace()
returns an array of floating-point numbers. However, you can change the output type by specifying the dtype
parameter. Here’s an example:
import numpy as npnp.linspace(1, 10, dtype=int)
This will generate an array of integers instead of floating-point numbers:
array([ 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 4, 4, 4, 4, 4, 5, 5, 5, 5, 5, 6, 6, 6, 6, 6, 7, 7, 7, 7, 7, 8, 8, 8, 8, 8, 9, 9, 9,
[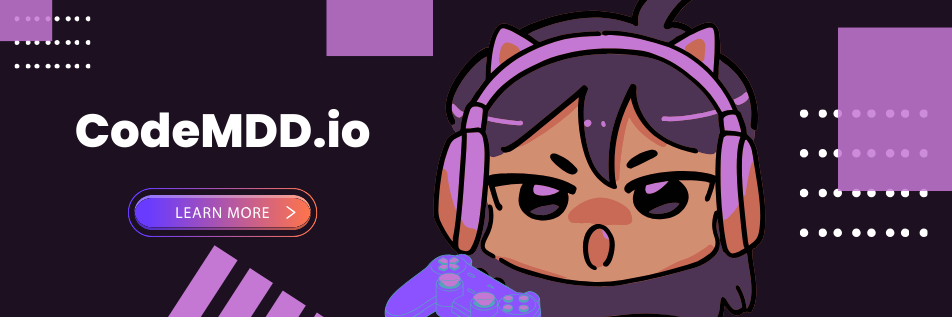](/python/)